Note
Go to the end to download the full example code
AnsMath sparse matrices and SciPy sparse matrices#
This example shows how to get AnsMath sparse matrices into SciPy sparse matrices.
Perform required imports and start PyAnsys#
Perform required imports.
from ansys.mapdl.core.examples import vmfiles
import matplotlib.pylab as plt
import ansys.math.core.math as pymath
# Start PyAnsys Math as a service.
mm = pymath.AnsMath()
Get matrices#
Run the input file from Verification Manual 153 and then
get the stiff (k
) matrix from the FULL file.
Copy AnsMath sparse matrix to SciPy CSR matrix and plot#
Copy the AnsMath sparse matrix to a SciPy CSR matrix. Then, plot the graph of the sparse matrix.
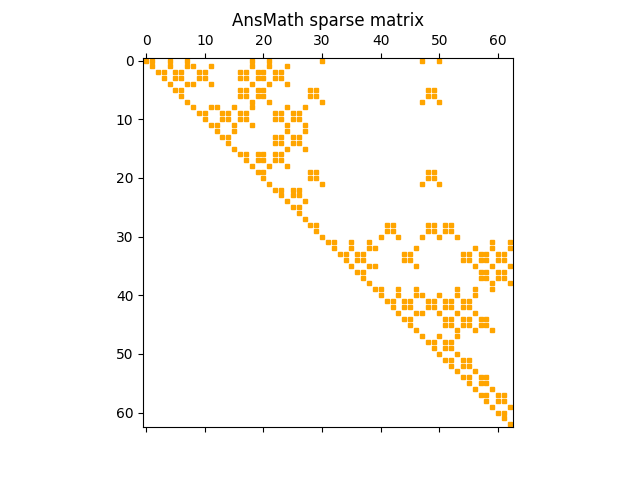
Access vectors#
You can access the three vectors that describe this sparse matrix with:
pk.data
pk.indices
pk.indptr
For more information, see SciPy’s class description for the CSR (compressed sparse row) matrix.
print(pk.data[:10])
print(pk.indices[:10])
print(pk.indptr[:10])
[ 0.20292539 0.00378143 -0.05003569 -0.01392161 -0.05003569 -0.01392161
-0.05142701 0.02406179 -0.05142701 0.20090661]
[ 0 1 4 7 18 21 30 47 50 1]
[ 0 9 17 29 40 47 57 66 72 79]
Create AnsMath sparse matrix from SciPy sparse CSR matrix#
Create an AnsMath sparse matrix from a SciPy sparse CSR matrix. Then, transfer the SciPy CSR matrix back to PyAnsys Math.
While this code uses a matrix that was originally within MAPDL, you can load any CSR matrix into PyAnsys Math.
my_mat = mm.matrix(pk, "my_mat", triu=True)
my_mat
AnsMath sparse matrix (63, 63)
Check that the matrices k
and my_mat
are exactly the sames. The
norm of the difference should be zero.
msub = k - my_mat
mm.norm(msub)
0.0
Print CSR representation in PyAnsys Math#
Printing the list of objects for the CSR representation in the PyAnsys Math space finds these objects:
Two SMAT objects, corresponding to the
k
,MSub
matrices, with encrypted names.The
my_mat
SMAT object. Its size is zero because the three vectors are stored separately.The three vectors of the CSR
my_mat
structure:MY_MAT_PTR
,MY_MAT_IND
, andMY_MAT_DATA
.
mm.status()
APDLMATH PARAMETER STATUS- ( 6 PARAMETERS DEFINED)
Name Type Mem. (MB) Dims Workspace
MY_MAT SMAT 0.000 [63:63] 1
VBHKYR SMAT 0.006 [63:63] 1
VXDWOF SMAT 0.006 [63:63] 1
MY_MAT_DATA VEC 0.003 344 1
MY_MAT_IND VEC 0.000 64 1
MY_MAT_PTR VEC 0.001 344 1
Access ID of Python object#
To determine which PyAnsys Math object corresponds to which Python object,
access the id
property of the Python object.
name(k)=VXDWOF
name(my_mat)=my_mat
name(msub)=VBHKYR
Stop PyAnsys Math#
Stop PyAnsys Math.
mm._mapdl.exit()
/opt/hostedtoolcache/Python/3.10.14/x64/lib/python3.10/site-packages/ansys/mapdl/core/launcher.py:811: UserWarning: The environment variable 'PYMAPDL_START_INSTANCE' is set, hence the argument 'start_instance' is overwritten.
warnings.warn(
Total running time of the script: (0 minutes 0.594 seconds)