Note
Go to the end to download the full example code.
Use PyAnsys Math to solve a dense matrix linear system#
This example shows how to use PyAnsys Math to solve a dense matrix linear system.
# Perform required imports and start PyAnsys
# ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
# Perform required imports.
import time
import matplotlib.pyplot as plt
import numpy.linalg as npl
import ansys.math.core.math as pymath
# Start PyAnsys Math as a server.
mm = pymath.AnsMath()
Allocate dense matrix#
Allocate a dense matrix in the MAPDL workspace.
Copy matrices as NumPy arrays#
Copy the matrices as NumPy arrays before they are modified by a factorization call.
Solve using PyAnsys Math#
Solve the dense matrix linear system using PyAnsys Math.
Solving a (1000 x 1000) dense linear system using PyAnsys Math...
Elapsed time to solve the linear system using PyAnsys Math: 0.03992319107055664 seconds
Get norm of solution#
Get the norm of the PyAnsys Math solution.
mm.norm(x)
1.000000000000001
Solve using NumPy#
Solve the dense matrix linear system using NumPy.
Solving a (1000 x 1000) dense linear system using NumPy...
Elapsed time to solve the linear system using NumPy: 0.0240781307220459 seconds
Plot elapsed times#
Plot the elapsed times for PyAnsys Math and Numpy to solve the dense matrix linear system.
max_time = max(pymath_time, numpy_time)
fig = plt.figure(figsize=(12, 10))
ax = plt.axes()
x = ["PyAnsys Math", "NumPy"]
y = [pymath_time, numpy_time]
plt.title("Elapsed time to solve the linear system")
plt.ylim([0, max_time + 0.2 * max_time])
plt.ylabel("Elapsed time (s)")
ax.bar(x, y, color="orange")
plt.show()
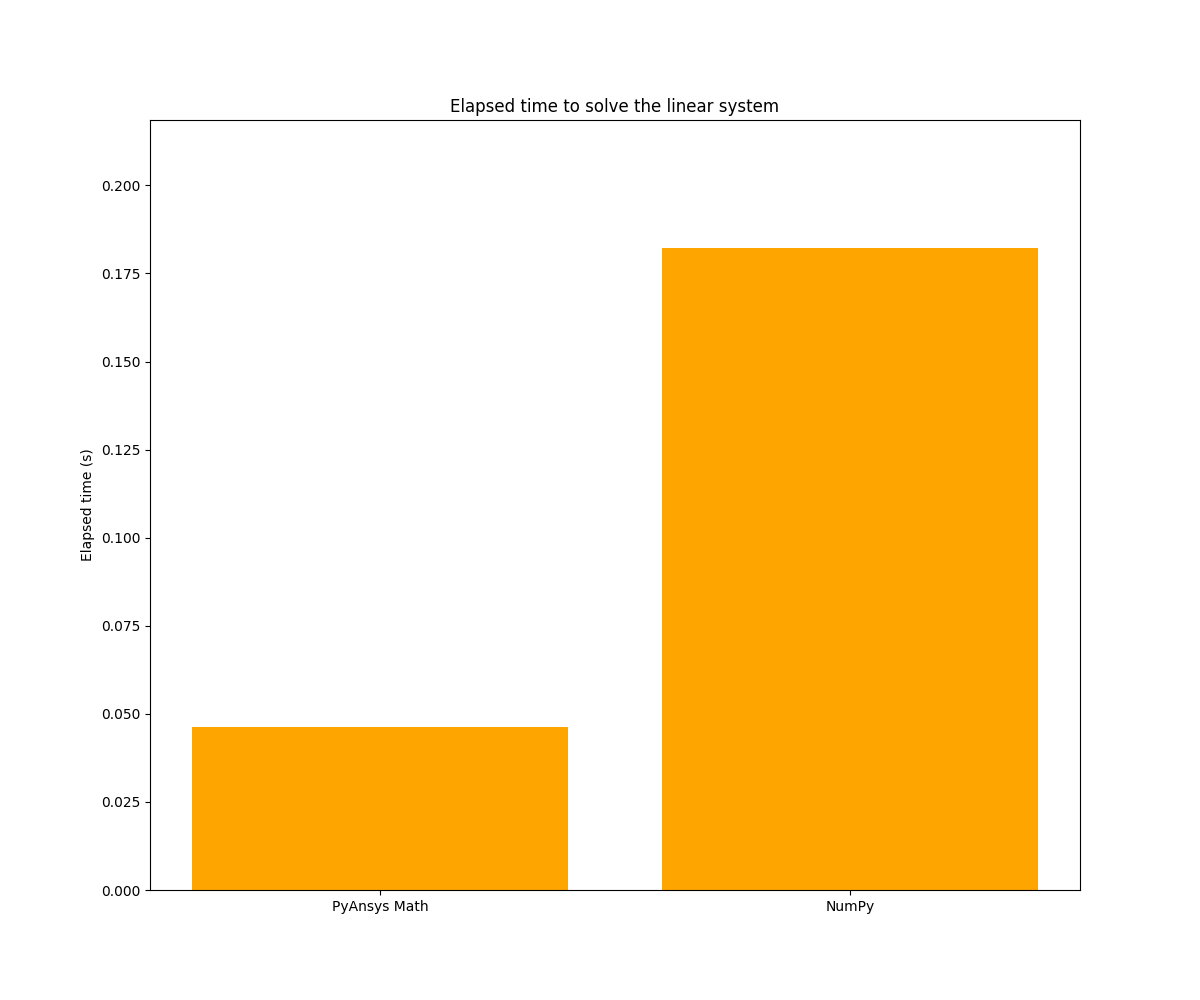
Get norm of solution#
Get the norm of the NumPy solution.
np.float64(0.9999999999999996)
Stop PyAnsys Math#
Stop PyAnsys Math.
mm._mapdl.exit()
Total running time of the script: (0 minutes 0.333 seconds)