Note
Go to the end to download the full example code.
Manipulate AnsMath vectors or dense matrices as NumPy arrays#
This example demonstrates how to use NumPy arrays to exchange data between PyAnsys Math and Python.
Note
This example requires Ansys 2021 R2 or later.
# Perform required imports and start PyAnsys
# ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
# Perform required imports.
import matplotlib.pyplot as plt
import numpy as np
import ansys.math.core.math as pymath
# Start PyAnsys Math as a server.
mm = pymath.AnsMath()
Convert AnsMath vector into NumPy array#
Allocate an AnsMath vector with 10 doubles.
apdl_vec = mm.ones(10)
print(apdl_vec)
ZWXSEL :
Size : 10
1.000e+00 1.000e+00 1.000e+00 1.000e+00 1.000e+00 < 5
1.000e+00 1.000e+00 1.000e+00 1.000e+00 1.000e+00 < 10
Create a NumPy array from this AnsMath vector.
Note that these are two separate objects. Memory is duplicated. Modifying one object does not modify its clone.
[1. 1. 1. 1. 1. 1. 1. 1. 1. 1.]
You can manipulate this NumPy array with all existing NumPy features.
[4. 4. 4. 4. 4. 4. 4. 4. 4. 4.]
Alternatively, the AnsMath object can be operated on directly with using NumPy methods.
print(np.max(apdl_vec))
print(np.linalg.norm(apdl_vec))
1.0
3.1622776601683795
Note that some methods have APDL corollaries, and these methods are more efficient if performed within PyAnsys Math.
For example, the norm method can be performed within PyAnsys Math.
print(apdl_vec.norm(), np.linalg.norm(apdl_vec))
3.1622776601683795 3.1622776601683795
Copy NumPy array to an AnsMath vector#
You can push back any NumPy vector or 2D array to PyAnsys Math. This
creates a new AnsMath vector, which in this case is named "NewVec:
.
mm.set_vec(pv, "NewVec")
print(mm.status())
APDLMATH PARAMETER STATUS- ( 2 PARAMETERS DEFINED)
Name Type Mem. (MB) Dims Workspace
NEWVEC VEC 0.000 10 1
ZWXSEL VEC 0.000 10 1
None
Create a Python handle to this vector#
Create a Python handle to this vector by specifying its name.
v2 = mm.vec(name="NewVec")
print(v2)
NEWVEC :
Size : 10
4.000e+00 4.000e+00 4.000e+00 4.000e+00 4.000e+00 < 5
4.000e+00 4.000e+00 4.000e+00 4.000e+00 4.000e+00 < 10
Apply same features to dense arrays#
You can apply the same features to dense APDL matrices and NumPy arrays.
Allocate an AnsMath dense matrix.
apdl_mat = mm.rand(3, 3)
plt.imshow(apdl_mat, cmap="YlOrBr")
plt.colorbar()
plt.title("AnsMath dense matrix")
plt.show()
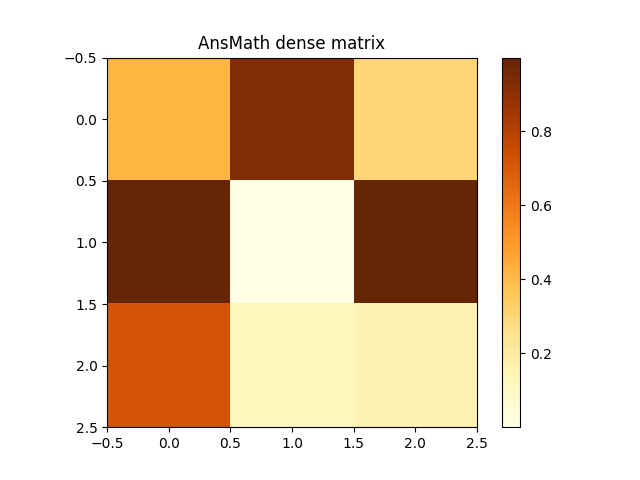
Convert the AnsMatch dense matrix to a NumPy array.
np_arr = apdl_mat.asarray()
assert np.allclose(apdl_mat, np_arr)
print(apdl_mat)
print(np_arr)
EMOZER:
[1,1]: 4.170e-01 [1,2]: 9.326e-01 [1,3]: 3.023e-01
[2,1]: 9.972e-01 [2,2]: 1.144e-04 [2,3]: 9.990e-01
[3,1]: 7.203e-01 [3,2]: 1.281e-01 [3,3]: 1.468e-01
[[4.17021999e-01 9.32557361e-01 3.02332568e-01]
[9.97184808e-01 1.14381197e-04 9.99040516e-01]
[7.20324489e-01 1.28124448e-01 1.46755893e-01]]
Use the matrix
method to load the NumPy array to APDL.
np_rand = np.random.random((4, 4))
ans_mat = mm.matrix(np_rand)
# Print the autogenerated name of this matrix.
print(ans_mat.id)
OEUHMY
Load this matrix from APDL and verify it is identical.
from_ans = ans_mat.asarray()
print(np.allclose(from_ans, np_rand))
True
Stop PyAnsys Math#
Stop PyAnsys Math.
mm._mapdl.exit()
/home/runner/work/pyansys-math/pyansys-math/.venv/lib/python3.10/site-packages/ansys/mapdl/core/launcher.py:818: UserWarning: The environment variable 'PYMAPDL_START_INSTANCE' is set, hence the argument 'start_instance' is overwritten.
warnings.warn(
Total running time of the script: (0 minutes 0.346 seconds)