Note
Go to the end to download the full example code.
Perform sparse factorization and solve operations#
Using PyAnsys Math, you can solve linear systems of equations based on sparse or dense matrices.
# Perform required imports and start PyAnsys
# ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
# Perform required imports.
from ansys.mapdl.core.examples import vmfiles
import matplotlib.pyplot as plt
import ansys.math.core.math as pymath
# Start PyAnsys Math as a server.
mm = pymath.AnsMath()
Factorize and solve sparse linear systems#
Run a MAPDL solve to create a Full file. This code uses a model from the official verification manual.
After a solve command, the FULL
file contains the assembled stiffness
matrix, mass matrix, and load vector.
List files in current directory#
List the files in current directory.
mm._mapdl.list_files()
['SCRATCH', 'TABLE_1', '__tmp_sys_out_xmkasqxrul__', 'anstmp', 'cleanup-ansys-1737e8cd23c7-548.sh', 'file.DSP', 'file.emat', 'file.esav', 'file.full', 'file.ldhi', 'file.mlv', 'file.mntr', 'file.mode', 'file.rdb', 'file.rst', 'file.rstp', 'file.stat', 'file0.DSP', 'file0.emat', 'file0.err', 'file0.esav', 'file0.full', 'file0.log', 'file0.mlv', 'file0.mode', 'file0.page', 'file0.r001', 'file0.rst', 'file000.jpg', 'file001.jpg', 'file002.jpg', 'file003.jpg', 'file004.jpg', 'file1.DSP', 'file1.emat', 'file1.err', 'file1.esav', 'file1.full', 'file1.log', 'file1.mlv', 'file1.mode', 'file1.out', 'file1.page', 'file1.r001', 'file1.rst', 'vm152.vrt', 'vm153.vrt']
Extract stiffness matrix#
Extract the stiffness matrix from the FULL file in a sparse
matrix format. For help on the stiff
function, use the
help(mm.stiff)
command.
Print dimensions of sparse matrix#
Print the dimensions of the sparse matrix.
AnsMath sparse matrix (27, 27)
Copy AnsMath sparse matrix to SciPy CSR matrix and plot#
Copy the AnsMath sparse matrix to a SciPy CSR matrix. Then, plot the graph of the sparse matrix.
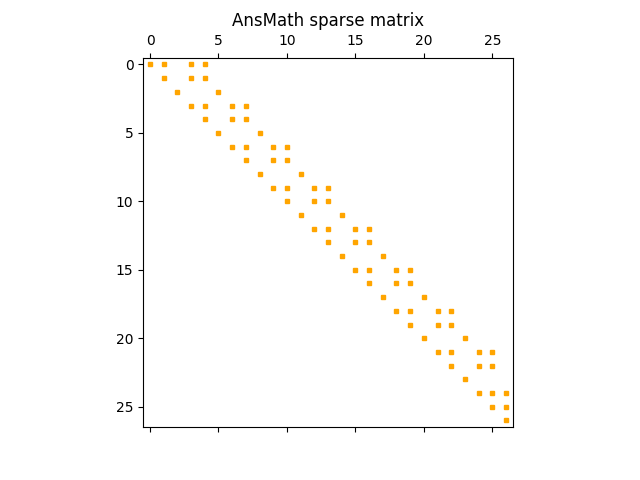
Get a copy of sparse matrix as a NumPy array#
Get a copy of the k
sparse matrix as a NumPy array
<Compressed Sparse Row sparse matrix of dtype 'float64'
with 77 stored elements and shape (27, 27)>
Extract load vector from FULL file and print norm#
Extract the load vector from the FULL file and print the norm of this vector.
b = mm.rhs(fname=fullfile, name="B")
b.norm()
0.12181823800246581
Get a copy of load vector as a NumPy array#
Get a copy of the load vector as a NumPy array.
by = b.asarray()
Factorize stiffness matrix#
Factorize the stiffness matrix using PyAnsys Math.
s = mm.factorize(k)
Solve linear system#
Solve the linear system.
x = s.solve(b)
Print norm** of solution vector#
Print the norm of the solution vector.
x.norm()
7.774533362578086e-06
Check accuracy of solution#
Check the accuracy of the solution by verifying that \(KX - B = 0\).
kx = k.dot(x)
kx -= b
print("Residual error:", kx.norm() / b.norm())
Residual error: 3.014095088656435e-16
Get a summary of allocated objects#
Get a summary of all allocated AnsMath objects.
mm.status()
APDLMATH PARAMETER STATUS- ( 5 PARAMETERS DEFINED)
Name Type Mem. (MB) Dims Workspace
K SMAT 0.001 [27:27] 1
B VEC 0.000 27 1
JSBVYU VEC 0.000 27 1
UHBYAG VEC 0.000 27 1
GYCMRY LSENGINE -- -- 1
Delete all AnsMath objects#
Delete all AnsMath objects.
mm.free()
Stop PyAnsys Math#
Stop PyAnsys Math.
mm._mapdl.exit()
/home/runner/work/pyansys-math/pyansys-math/.venv/lib/python3.10/site-packages/ansys/mapdl/core/launcher.py:822: UserWarning: The environment variable 'PYMAPDL_START_INSTANCE' is set, hence the argument 'start_instance' is overwritten.
warnings.warn(
Total running time of the script: (0 minutes 0.612 seconds)